Task: The Level Streaming
02.05.2010

Softwares:

In Contre-Jour, the levels are short and the player ends them quickly. In order to gain time in loading the scenes, I created a level streaming manager.
At the game start, the manager loads the current level where the player characeter is and lanches the game. Next, the level is playable directly but during the game, the manager continues and load the levels directly linked to the one we are playing. For example, when the hero is in the first map ( the red point), the second level is preloaded.
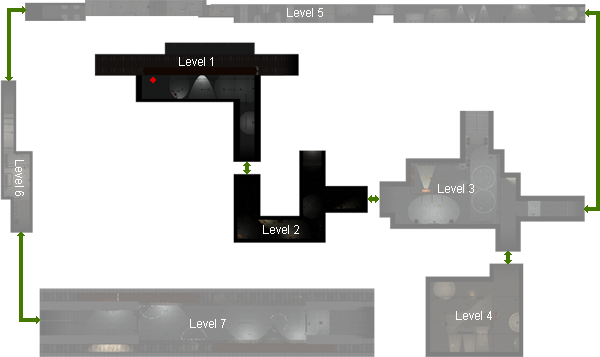
If the level 2 is loaded when the player finishes the first then we go to that level without any loading screen. Arrived in this new place the third one is loading and so on. The first map stays loaded because it's directly linked. We can go back without waiting.
On the code side
The level streaming manager uses a list of all the levels. Each level item is made of a scene to load and a boolean list.
- isLoading: Is the level currently loading
- isDataLoaded: Is the level loaded
[System.Serializable]
public class levelsList{
public string name;//level identifier
public string description;
[System.NonSerialized]
public bool isLoading = false;//Is the level loading
[System.NonSerialized]
public bool isDataLoaded = false;//Is the level loaded
}
public class GameManager : MonoBehaviour {
public LevelsListElement[] levelsList;//List of levels
//...
}
Unity3D contains a simple function to load a scene in asynchronous mode.
private IEnumerator streamLevelCoroutine(int level){
if(!levelsList[level].isLoading && !levelsList[level].isDataLoaded){//We load the level only if needed
//LoadLevelAdditiveAsync: Stream the loading of a scene.
yield return Application.LoadLevelAdditiveAsync(levelsList[level].name);
levelsList[level].isDataLoaded = true;//The level is loaded and available
levelsList[level].isLoading = false;//Loading ends
}
}
Summary
To make a quality game, we decided to minor the time of the loading screen between the levels.
Work: I coded a level streaming manager.