My skills / ActionScript

Flash was a graphic software at first and interactions were limited. Later, it changed in a true engine allowing to create softwares and video games on the web. Now, ActionScript (version 3) became a huge programming language that proposes a lot of features.
My skills
- ActionScript 2: 2 years in companies (softwares, games)
- ActionScript 3: 1 year for personal use (videogames)
- hAxE (meta-language based on ActionScript): Some experiments
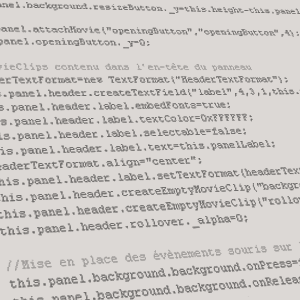
Task: Synchronization: Frame/Music
08.12.2010

Softwares:

On Pump it! I had to code the synchronization managment between the game and the music.
The deltaTime
Usually, real-time videogames work on the deltaTime purpose: it's the time difference between two successive frames. Normally, this time should be steady:
For an application in 30 frames per second, the time between two frames is 1/30 a second: 0.03333.. s.

Nevertheless, according to the hardware, to the processor activity, this time fluctuates during the execution. Know it is useful to move the screen elements in order to fill the framerate slowdowns or increases. The game is fluid then and even when the framerate is low, the time remains constant.
Synchronization with the music
To synchronize the game with the music, all we have to do is to calculate the deltaTime using the audio track. That means we calculate the difference between the readhead position in milliseconds between the current frame and the previous one. Then, if the audio track slows down or plays faster, the elements positions on the screen are adjusted and perfectly synchronized to the sound.

On the code side
We calculate the deltaTime corresponding to the sound track with a function called on each frame.
import flash.display.MovieClip;
import flash.events.Event;
import flash.media.SoundChannel;
public final class SoundTimer
{
private var _channel:SoundChannel;//A reference to the music
private var _lastTime:Number;//Keep the readhead position on the last frame
private var _deltaTime:Number = 0;//Keep the deltaTime
public static function get deltaTime() {//the deltaTime can be reach anywhere
return _deltaTime;
}
public function SoundTimer(soundChannel:SoundChannel)
{
//We call updateDeltaTime on each OnEnterFrame
//updateDeltaTime is called on each frame
var mc:MovieClip = new MovieClip();
mc.addEventListener(Event.ENTER_FRAME, updateDeltaTime);
_lastTime = 0;//At start, the last time is 0
assignSound(soundChannel);//assign the reference to the music
}
public function assignSound(soundChannel:SoundChannel) {
channel = soundChannel;
}
private function updateDeltaTime(ev:Event) {
var currentTime:Number = _channel.position;//We get the readhead position on the current frame
_deltaTime = (currentTime-_lastTime) / 1000;//we get the difference between the current frame and the previous one
//The position at the previous frame is changed by the current position
//Then, when the function is called on the next frame, the previous position is the previous frame value.
_lastTime = currentTime;
}
}
Next, we use the deltaTime to calculate the notes movement, for example.
//! This code isn't optimized but it illustrate the example
//...
private var tones:Vector.<Sprite>//Keep the tones
private tempo:Number = 120;//The tempo in beats/minuts is regular here
private laneWidth:Number = 200;//The length in pixels travelled by a tone on each beat
//...
//Update is called on each frame
public function update() {
for each(var tone:Sprite in tones) {
//We translate the tempo in seconds
//We multiply by the length it must travel in a beat
//We multiply by the time elapsed between the current and the previous frame to get synchronize with the music
tone.x += tempo / 60 * laneWidth * SoundTimer.deltaTime;
}
}
//...
Summary
The main feature of the game is the synchro between music and display.
Responsability: Coding the feature.